Running React with Rails 7
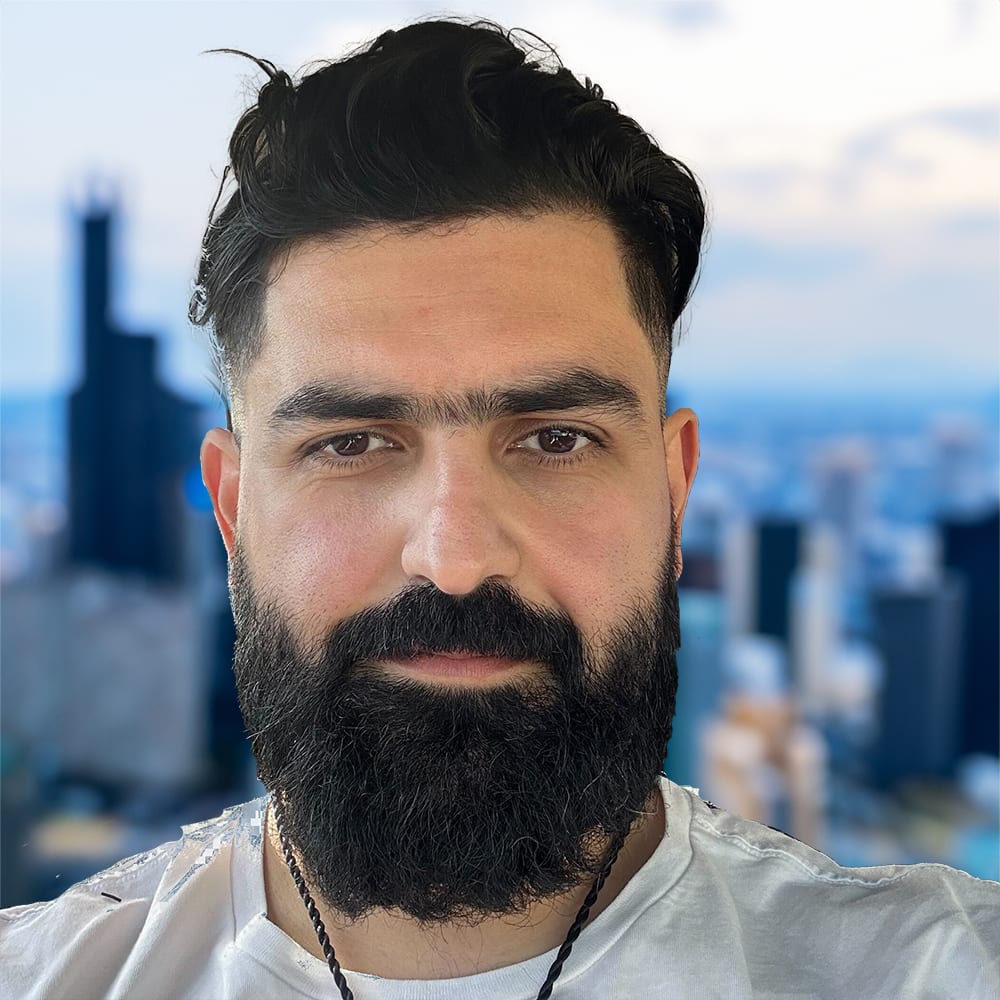
Importmap Introduction
In web and software development, Rails 7 has brought a wave of innovation, especially within the JavaScript integration field. Something to highlight in regards to this is the introduction of Importmap, created by Sam Stephenson and supported by David Heinemeier Hansson himself. Importmap isn’t an add-on optional feature but is now default for new Rails projects. It has been proven that it is possible to seamlessly integrate React into Rails using the Importmap even without the conventional .jsx extension. This paradigm assures a more intuitive and smooth-running experience for JavaScript development when working in Rails ecosystem.
React integration to Rails 7 with testing tools
We will demonstrate an integration of React within Rails ecosystem with Rails 7 by creating a simple and classic “Hello World” app that we will also be integrating with Jest for testing purposes. As we should al know, testing is a very important part of Front-End development as in every aspect of development such as Back-End.
Create a Rails app:
We can start of by creating our Rails app
$ rails new hello_world_project -j=esbuild
This is a usual step when starting a new Rails project, but to add React, it doesn’t take that much difference thanks to Importmap
Install react and react-dom:
$ cd hello_world_project && npm i react react-dom
This way we have the old tools and environment from React.
Create a file in: app/javascript/react/src/components/Hello.jsx:
import * as React from 'react'
import * as ReactDOM from 'react-dom'
const Hello = () => {
return (Hello, Rails 7!)
}
// Use it if you don't plan to use "remount"
// document.addEventListener('DOMContentLoaded', () => {
// ReactDOM.render( , document.getElementById('hello'))
// })
export default Hello
Keep in mind that remounting is used to reset state, values, and data and it also lets you use your React components just like any other HTML element, meaning that you can use React with other DOM library/framework like Vue, Angular, etc.
We will use remount so we can render our components using simple HTML tags wherever in the project. ### Install remount:
$ npm i remount
Create a file in: app/javascript/react/src/index.js:
In here we can then map our components to the HTML tags we want and we also define the component by using the Hello component we imported.
import { define } from 'remount'
import Hello from "./components/Hello"
define({ 'hello-component': Hello })
Import the React index in: app/javascript/application.js:
import "./react/src/index.js"
We can then generate a controller so we can test if our integration is actually working.
Generate a controller for test purposes:
$ rails g controller hello_world
Import our component in app/views/hello_world.html.erb:
<h1>Hello#world</h1>
<hello-component/>
Time to run our app:
In this case, we don’t run the app by using the classic bash rails server
or bash rails s
, we run this app with:
$ ./bin/dev
Now you can see our component in http://localhost:3000/hello_world and out component works as expected in a Rails 7 ecosystem
Testing our component with Jest
As we stated previously, testing is a very important task we have to complete when creating an app and each component to ensure it works in every case that may comes up when the app is running and with all elements that we have created for it working together or individually.
For this to be possible, we need to install Jest (testing tool).
Install jest and dependencies:
$ npm install — save-dev jest babel-jest @babel/preset-env @babel/preset-react react-test-renderer
Create a babel.config.js in the root rails app with:
module.exports = {
presets: [‘@babel/preset-env’, ‘@babel/preset-react’],
};
Now, write the tests in app/javascript/react/src/components/Hello.test.jsx:
import React from 'react'
import renderer from 'react-test-renderer';
import Hello from './Hello';
test('My test', () => {
// Write tests here
});
We can finally after all these steps, run the app for testing by using:
bash npm run test
Conclusion
In summary, React setup within Rails 7 has been enriched by the introduction of Importmap which tells us that integration of JavaScript technologies in Rails environments will be more significant and easier as it progresses. Importmap’s streamlined approach allows developers to incorporate React components in a easy and smooth way into Rails applications, even without the usual .jsx extension.